Using PHP Traits the Right Way: Cleaner Code, Less Repetition
- vartikassharmaa
- May 3
- 3 min read
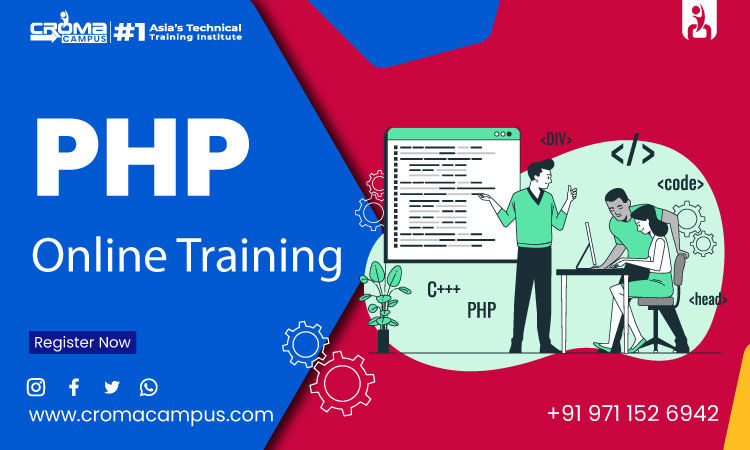
Introduction:
Writing code is simple. Writing neat, reusable code isn't. That's where Traits come in handy. They're an intelligent solution to preventing redundant functions.
Most students learn Traits during hands-on practice in a PHP Training Course in Noida. It's a hands-on skill that addresses actual coding problems.
If you're applying inheritance to all your shared methods, your code will start to become a mess. Traits provide you with an alternative. They're like sets of reusable methods. And they can be used between unrelated classes. That's powerful.
What Traits Do and Why They Matter?
A Trait is a set of methods. No copying the method repeatedly. PHP doesn't support multiple inheritance. Traits come to your rescue.
For example:
trait Logger {
public function log($msg) {
echo "Log: " . $msg;
}
}
class User {
use Logger;
}
The User class can now use the log() function. No need to extend any class. Traits help teams write less code. They keep logic in one place.
In places like Noida, many dev teams use Traits in microservices. It’s a common part of project work during PHP Training in Delhi sessions.
Using Multiple Traits Together:
That makes them flexible.
Let’s say Trait A and Trait B both have hello().
trait A {
public function hello() {
echo "Hello from A";
}
}
trait B {
public function hello() {
echo "Hello from B";
}
}
class MyClass {
use A, B {
B::hello insteadof A;
A::hello as helloA;
}
}
Here, we chose B’s version of hello(). We also kept A’s version under a new name, helloA.
This solves the method conflict. It also adds more control.
This is taught early in advanced lessons during PHP Online Training. Many new devs make mistakes when using multiple Traits. This trick helps avoid bugs.
When Not to Use Traits:
Traits are helpful. But they should be used carefully. Don't treat them like garbage bags for all helper methods.
Here’s when you should avoid Traits:
● When the logic needs object states or properties.
● When the Trait has unrelated methods.
● When too many Traits make your class unreadable.
Traits should not hold business logic. Keep them clean and focused.
In many PHP Training in Delhi workshops, developers are told to use Traits only for logic that’s generic. Like logging, file handling, or API formatting.
If the Trait starts doing too much, break it down.
How to Structure and Name Traits?
Traits are reusable tools. Like all tools, they must be organized.
Use clear names. Like LoggerTrait, EmailHelperTrait, or CacheTrait. This makes their purpose clear.
Also, follow best practices:
Best Practice | Why It Matters |
Name clearly | So it's easy to know what the Trait does |
Keep it short | Avoid Traits with 20+ methods |
Avoid properties | Traits should not manage class state |
Document it | Use PHPDoc to describe what the Trait is for |
Limit count | Using more than 3 Traits in one class makes it confusing |
Some developers learning via an Online PHP Course with Certificate also combine Traits with interfaces. This adds structure to the design.
In Noida, many project-based roles look for devs who can build modular apps.
Advanced Uses of Traits:
You can also use abstract methods inside a Trait. This forces the class to define them.
trait APIClient {
abstract public function getToken();
public function sendRequest($data) {
$token = $this->getToken();
// send request with token
}
}
Now, any class using APIClient must define getToken(). This pattern is powerful. It ensures the class follows a rule.
Traits also support private methods. These can help split logic inside the Trait without exposing it to the class.
You can even nest Traits inside Traits using one Trait inside another Trait. But avoid overusing this.
Traits also don’t support constructors. So you can’t initialize data inside them.
These points are often skipped in beginner content. But mastering these tricks gives you better control. That’s why mentors in the PHP Training Course in Noida now include small Trait-based problems in exams.
Sum Up:
Traits help reduce repetition in PHP. They solve the problem of single inheritance. Use instead of and as to manage conflicts. Don’t overload Traits with unrelated functions. Use clear names and follow best practices. Traits should be part of your code planning, not a shortcut.
Comments